初めてのWPF 9日目
2019/01/26
やりたいこと
起動時に押せるボタン:ファイルオープン、CSVインポート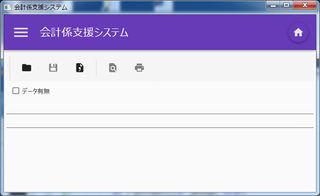
データ入力後に押せるボタン:保存、印刷プレビュー、印刷
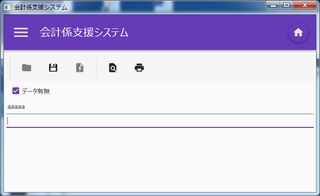
データを空にすると起動時と同じ状態
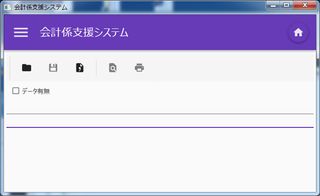
MaterialDesignToolBarを使う
View側は楽勝。TextBoxやCheckBoxはスタイルの指定すら不要。ToolBarのRegionをどうするかで迷いましたが、とりあえずModule側の基本画面に表示することに。
<ToolBarTray DockPanel.Dock="Top"> <ToolBar Style="{DynamicResource MaterialDesignToolBar}" ClipToBounds="True"> <Button ToolTip="ファイルを開く" Command="{Binding FileOpenCommand}"> <materialDesign:PackIcon Kind="Folder" /> </Button> <Button ToolTip="データを保存" Command="{Binding FileSaveCommand}"> <materialDesign:PackIcon Kind="Floppy" /> </Button> <Button ToolTip="CSVファイルをインポート" Command="{Binding CsvImportCommand}"> <materialDesign:PackIcon Kind="FileDelimited" /> </Button> <Separator /> <Button ToolTip="印刷プレビュー" Command="{Binding PrintPreviewCommand}"> <materialDesign:PackIcon Kind="FileFind" /> </Button> <Button ToolTip="印刷" Command="{Binding PrintCommand}"> <materialDesign:PackIcon Kind="Printer" /> </Button> </ToolBar> </ToolBarTray>
DelegateCommandを使う
NameSpace追加
using Prism.Commands;
アイコンの数だけDelegateCommand??
public DelegateCommand FileOpenCommand { get; private set; } public DelegateCommand FileSaveCommand { get; private set; } public DelegateCommand CsvImportCommand { get; private set; } public DelegateCommand PrintPreviewCommand { get; private set; } public DelegateCommand PrintCommand { get; private set; }
ボタンのCanExecuteを制御
チェックボックスで制御するのはサンプル通りなので直ぐに出来ましたが、保存・印刷可能なコンテンツがあるかどうかで制御したいわけでTextBoxが空かどうかで制御しようとしたらどうしたらいいのか分からず。テキストボックスの値が変わった時にRaiseCanExecuteChangedを呼んでも動きましたが、最終的にはObservesPropertyを使ってとりあえず動いたよ。
// bootをプロパティに変える private bool HaveData() { return !string.IsNullOrEmpty(InputData); } private bool NoData() { // 微妙ですが… return string.IsNullOrEmpty(InputData); } public DelegateCommand FileOpenCommand { get; private set; } public DelegateCommand FileSaveCommand { get; private set; } public DelegateCommand CsvImportCommand { get; private set; } public DelegateCommand PrintPreviewCommand { get; private set; } public DelegateCommand PrintCommand { get; private set; } public CashbookViewModel() { // ObservesPropertyでInputDataの変化を監視し、NoDataを再評価する感じ? FileOpenCommand = new DelegateCommand(FileOpen, NoData).ObservesProperty(() => InputData); FileSaveCommand = new DelegateCommand(FileSave, HaveData).ObservesProperty(() => InputData); CsvImportCommand = new DelegateCommand(CsvImport, NoData).ObservesProperty(() => InputData); PrintPreviewCommand = new DelegateCommand(PrintPreview, HaveData).ObservesProperty(() => InputData); PrintCommand = new DelegateCommand(Print, HaveData).ObservesProperty(() => InputData); }監視対象のフィールドが複数ある場合はチェインでつなぐことも可能なようです。